INTRODUCING ODIN
Voice chat for your apps, games and websites
Experience unparalleled audio quality and seamless integration with our next-generation Voice Chat SDK, including proximity chat for immersive, directly integrated 3D voice experiences. Designed for Unity, Unreal Engine, and even your favorite web browser, our SDK offers noise suppression, ultra-low-latency, and scalable architecture with both hosting and self-hosting options. Sync text messages, documents, or game states effortlessly using our versatile data channel. Transform your gaming and communication experience today.
Made for Developers
In just a few lines of code you can connect and join a room. See the full list of our SDKs here.
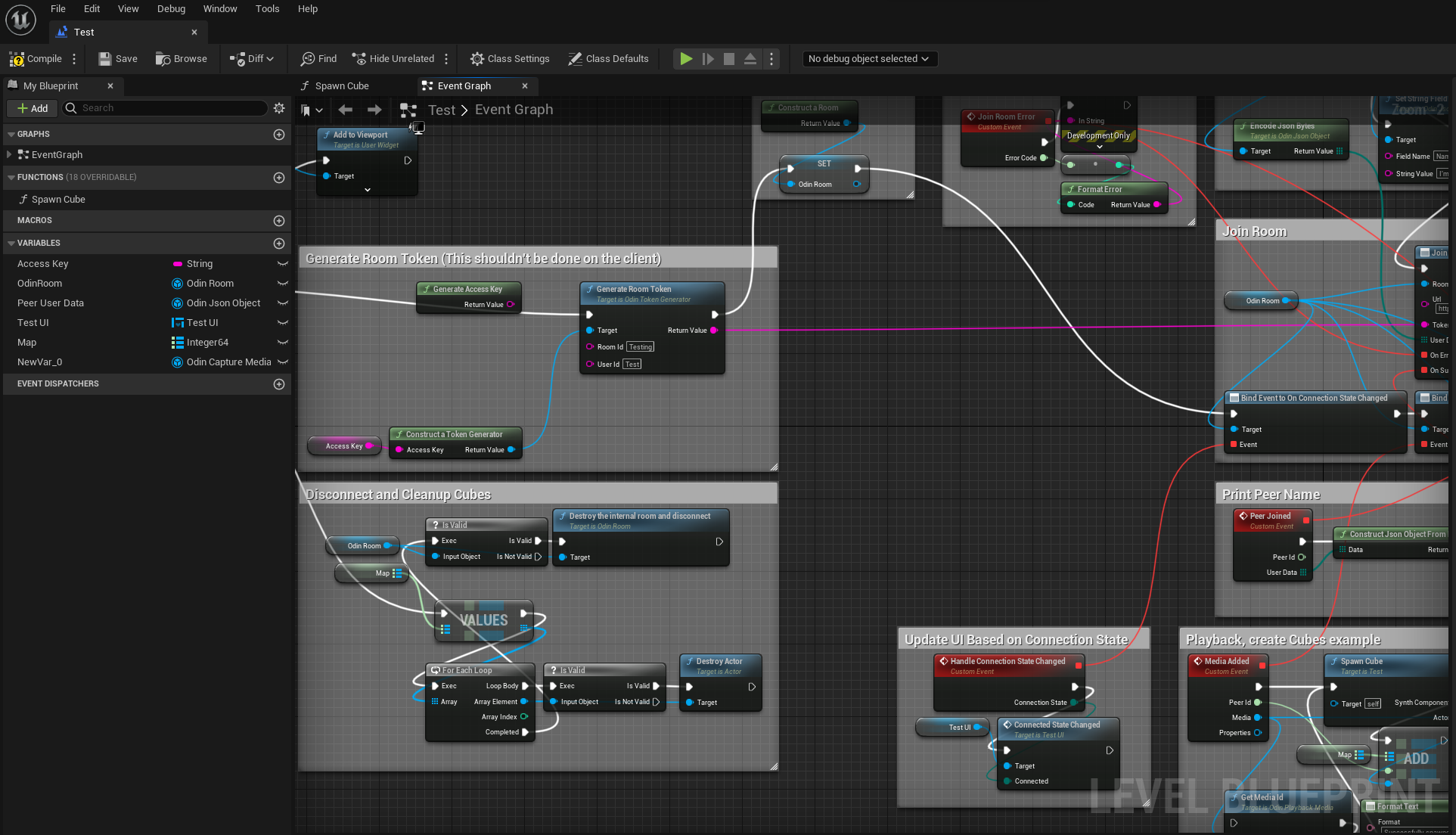
Manage all aspects of voice within the Unreal Engine Editor and integrate it quickly into your game or XR experience. Our ODIN SDK leverages Unreal Engines audio processing and is deeply integrated into Unreal Engine 4 (UE4) as well as Unreal Engine 5 (UE5) and supports Blueprints and automatic proximity chat (3D spatial audio).
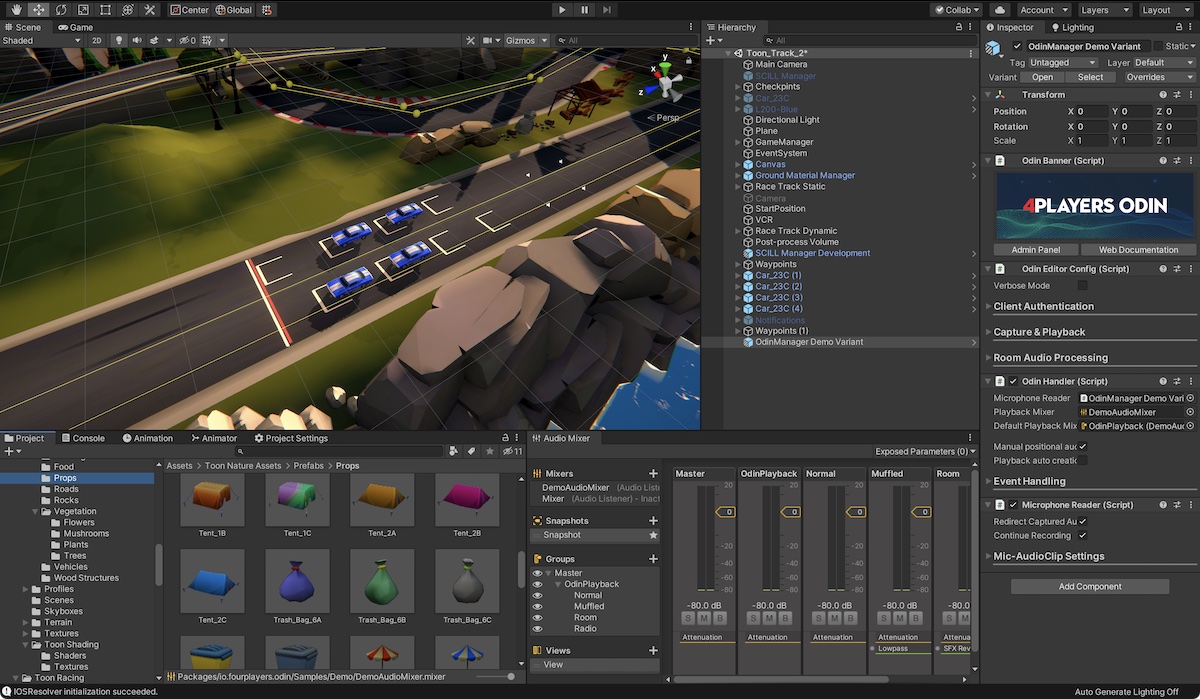
Manage all aspects of voice within Unity and integrate it quickly into your game or XR experience. Our ODIN SDK is deeply integrated into Unity and features automatic proximity chat (3D spatial audio). Use the tools you know, like AudioSource
, AudioListener
or the built-in Unity AudioMixer
, for your voice chat integration.
Add ODIN to your C or C++ projects with our simple to use ODIN Core SDK. Our Core SDK is the foundation of all other SDKs and features an easy-to-use interface.
// Generate access token - should be done on a server
char room_token[512];
OdinTokenGenerator* generator = odin_token_generator_create("__YOUR_ACCESS_KEY__");
odin_token_generator_create_token(generator, "My Room", "John Doe", room_token, sizeof(room_token));
odin_token_generator_destroy(generator);
// Setup event handler and join a room.
OdinRoomHandle room = odin_room_create();
odin_room_set_event_callback(room, handle_odin_event, NULL);
odin_room_join(room, gateway_url, room_token);
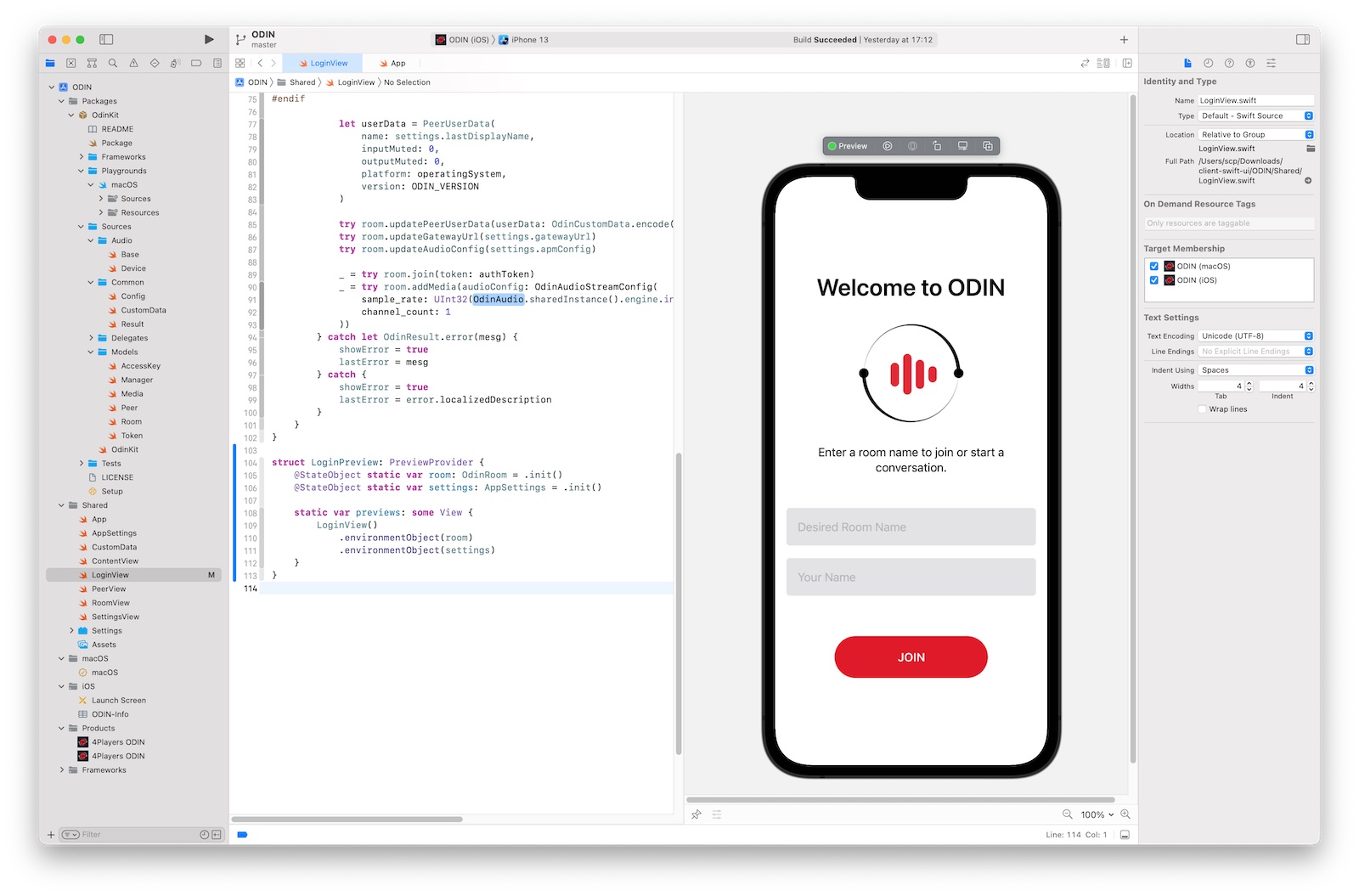
Manage all aspects of voice within Xcode and integrate it in quickly into your game or XR experience. Add real-time voice communication and text messaging in no time to your iOS and macOS apps or games. Our SDK is built on top of Apple’s Audio implementation and features automatic 3D spatial audio.
Integrating our Voice SDK in any website with vanilla JavaScript is seamless, effortless, and easy. Add our script and join a room, that’s it.
<html>
<head>
<script type="text/javascript" src="https://4npcdn.com/2355/odin/javascript/latest/odin.min.js"></script>
<script type="text/javascript">
var connectOdin = async function(room, user) {
const token = await getToken(room, user);
const odinRoom = await ODIN.OdinClient.initRoom(token);
await odinRoom.join();
return odinRoom;
}
connectOdin("My Room", "John Doe").then(odinRoom => {
console.log("Connected to room", odinRoom);
});
</script>
</head>
</html>
Use our NodeJS ODIN SDK to create AI bots or scripts that interacts with your game or XR experience. Useful for content moderation, analytics, recording and AI enhanced human interactions.
// Load the odin module and other libs
import odin from '@4players/odin-nodejs';
const {OdinClient} = odin;
const odinClient = new OdinClient();
const room = odinClient.createRoom("__YOUR_ACCESS_KEY__", roomName, userName);
// Send a message to the room using a JSON object (can be anything you like)
const sendMessage = (text) => {
const message = {
kind: 'message',
payload: text
}
room.sendMessage(new TextEncoder().encode(JSON.stringify(message)));
}
// Listen on PeerJoined messages and send a text message into the room
room.addEventListener('PeerJoined', (event) => {
sendMessage(`${event.userId} joined the room.`);
});
// Listen on PeerLeft messages and send a text message into the room
room.addEventListener('PeerLeft', (event) => {
sendMessage(`${event.userId} has left the room.`);
});
// Join the room and start receiving events
room.join("gateway.odin.4players.io");
Adding our Voice SDK to any modern JavaScript framework like Angular
, Svelve
, Vue
, React
is super easy. Just add our npm package @4players/odin
to your package.json and connect a room. That’s it.
import { OdinClient } from '@4players/odin';
import { TokenGenerator } from "@4players/odin-tokens";
const generator = new TokenGenerator(apiKey);
const token = generator.createToken("My Room", "John Doe");
const odinRoom = await OdinClient.initRoom(token);
await odinRoom.join();
Proximity Voice Chat
ODIN integrates seamlessly into Unity and Unreal Engine and provides native and easy to implement 3D proximity chat with audio occlusion.
Massively Scalable
ODIN supports hundreds of concurrent users in the same room with automated partitioning of data packets based on position and super efficent servers.
Features
Deep Game Engine Integration
ODIN is integrated directly into Unity and Unreal Engine 4 and 5, offering a seamless and powerful integration into new and existing apps, games and applications.
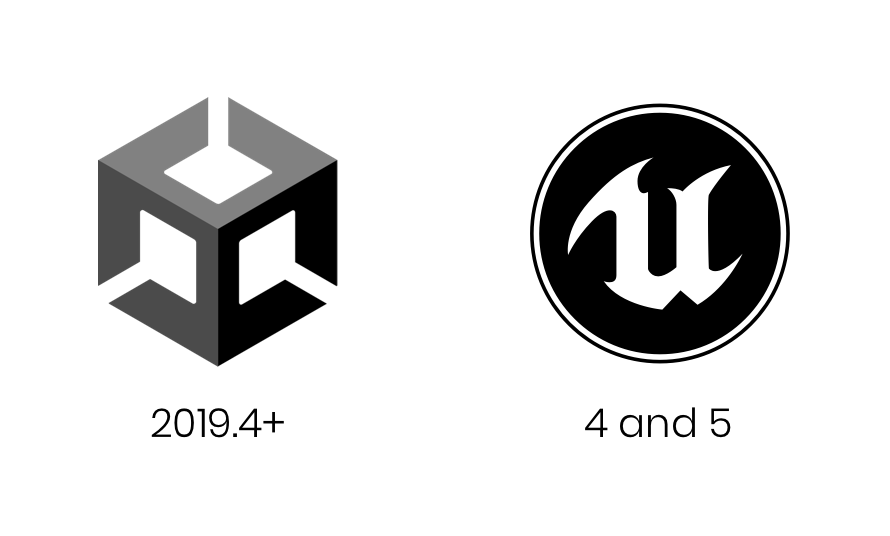
Host Yourself or Get Hosted
Our software runs in the highly optimized 4Players Cloud or you can host servers yourself in any cloud you want or on-premise on your own hardware.
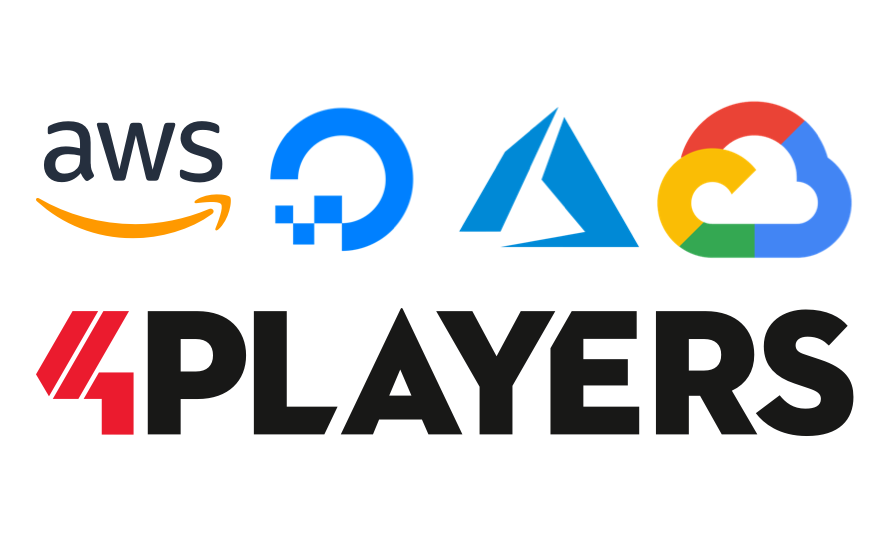
… and much more
Outstanding Voice Quality
Our proprietary technology based on latest internet technologies like QUIC/HTTP3 is built specifically for voice and low-latency data synchronization.
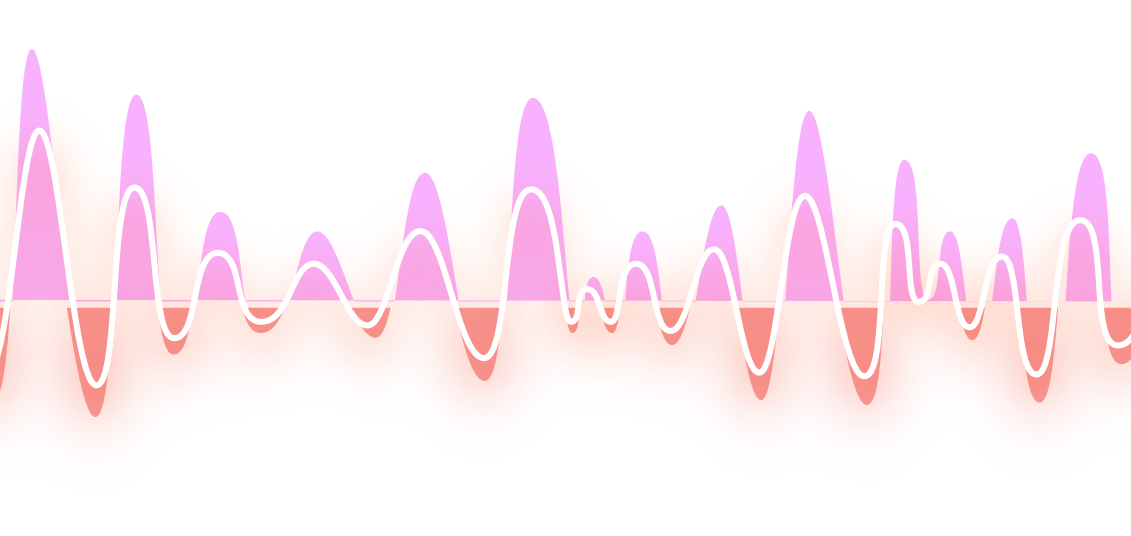
Real-Time Data and Networking
Unlike comparable tools, ODIN is integrated directly into existing game engines, offering a seamless and powerful connection.
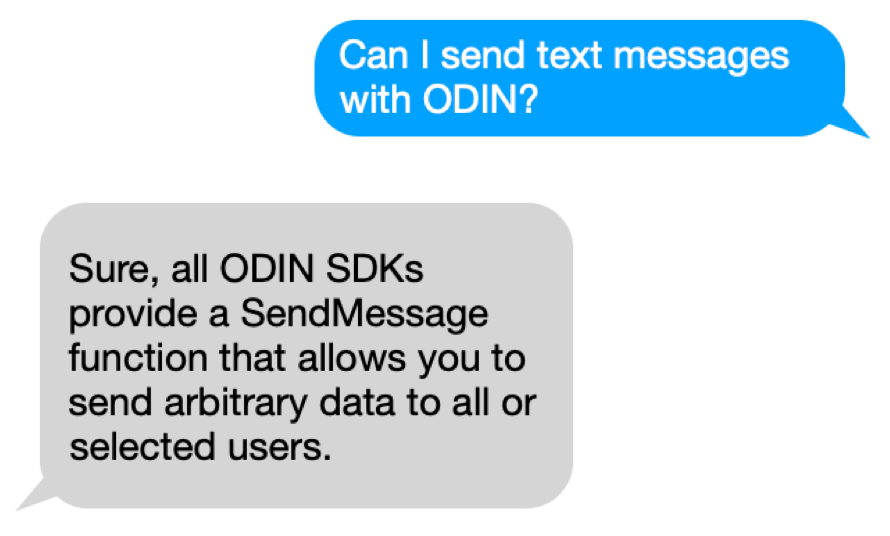
Native Apple Swift SDK
Implement real-time voice in your macOS, iOS, tvOS and watchOS apps with our native Swift SDK. It’s easy to use and integrates seamlessly into your existing code.
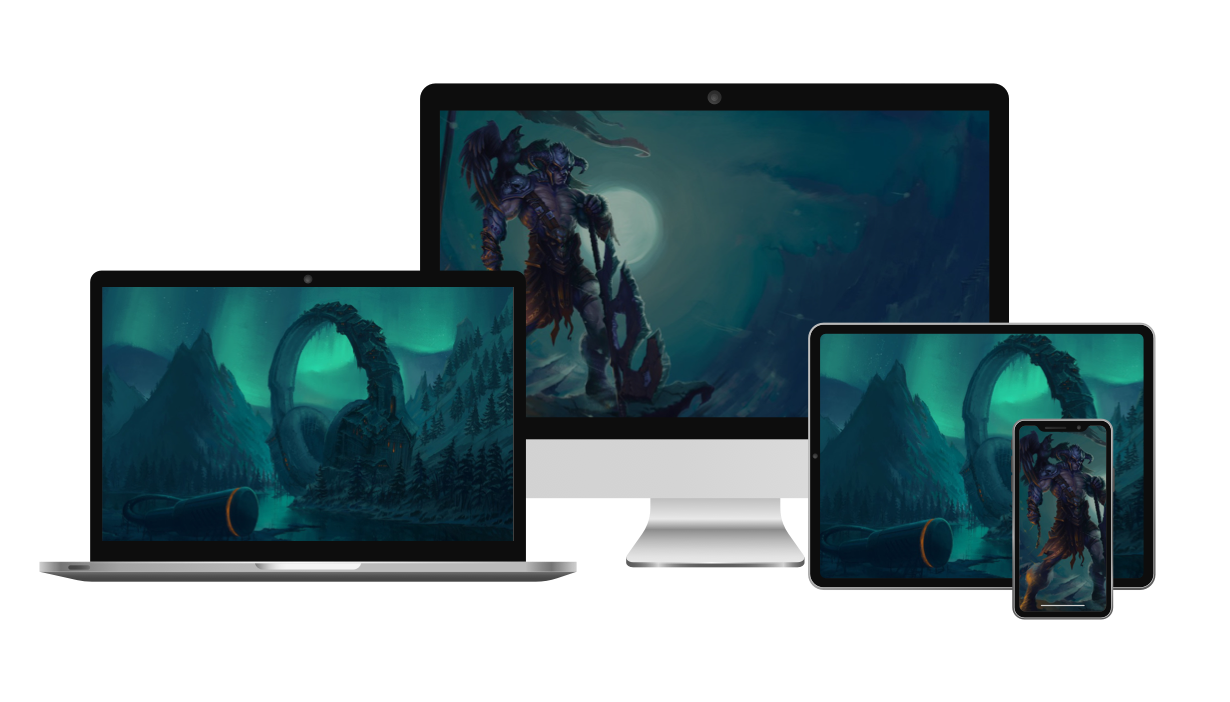
GDPR Compliant
Privacy and security is very important to us. ODIN is completely user agnostic and we don’t harvest any data or tell you how things should work or look. You are in complete control.
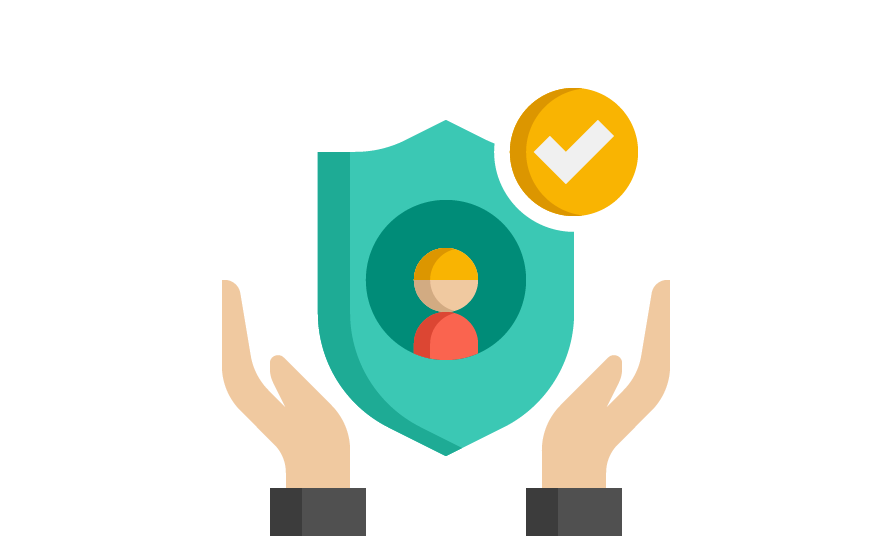
Horizontally Scalable
Our server is a single binary that can be scaled horizontally with ease using standard container orchestration software like Kubernetes, ECS or Openshift.
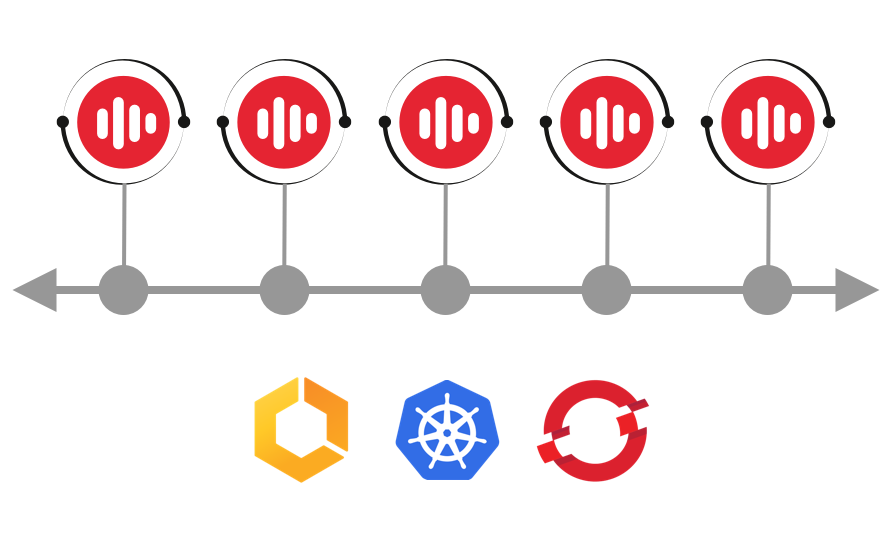
Web SDK
We provide a JavaScript/TypeScript SDK that can be used to integrate ODIN into any web application or website and that is even cross-platform compatible with your Unity or Unreal Engine app.
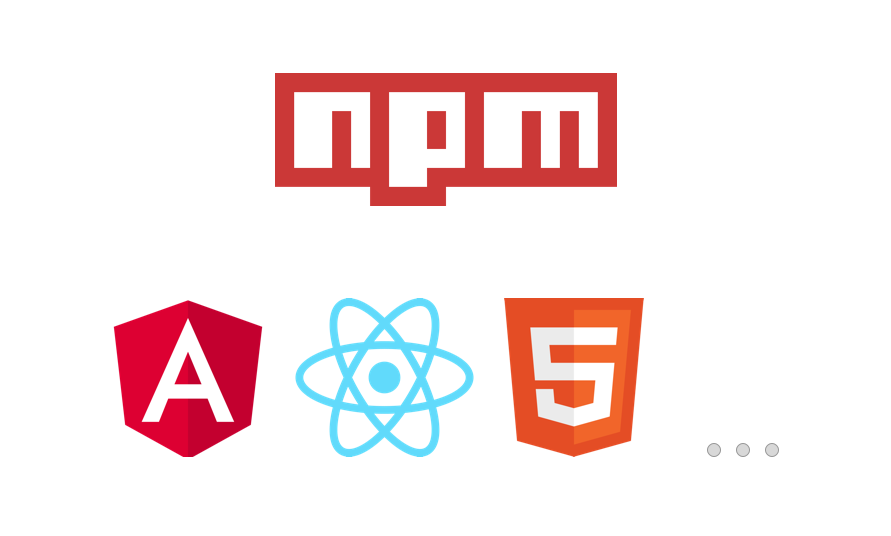
Odin’s Competitive Edge
Discover how ODIN stands out among the top voice SDKs in the industry.
ODIN | Photon | TeamSpeak | Agora | Vivox | EOS | |
---|---|---|---|---|---|---|
Hosting & Pricing | ||||||
✔ | ✔ | ✕ | ✔ | ✔ | ✔ | |
✔ | ✔ | ✔ | ✕ | ✕ | ✔ | |
✔ | ✕ | ✕ | ✕ | ✔ | ✔ | |
2000+ | 50 | 1000 | 128 | 16 | ||
Platform & Engine Integration | ||||||
✔ | ✔ | ✕ | ✔ | ✕ | ✕ | |
✔ | ✔ | ✕ | ✕ | ✕ | ✔ | |
✔ | ✕ | ✕ | ✕ | ✕ | ✔ | |
✔ | ✕ | ✕ | ✔ | ✕ | ✕ | |
✔ | ✕ | ✕ | ✔ | ✕ | ✕ | |
Core Communication Features | ||||||
✔ | ✕ | ✔ | ✔ | ✔ | ✕ | |
✔ | ✔ | ✔ | ✔ | ✔ | ✔ | |
✔ | ✔ | ✕ | ✕ | ✕ | ✕ | |
✔ | ✕ | ✔ | ✔ | ✔ | ✕ | |
✔ | ✕ | ✕ | ✕ | ✕ | ✕ | |
✔ | ✔ | ✕ | ✕ | ✕ | ✔ |
Supported Platforms
Different platforms, same code, equal performance. We support all major platforms, including iOS, Android, Windows, Mac, Linux, and more.
Pricing
Leverage the global reach of the 4Players Cloud, spanning over 14 locations worldwide. Our intelligent SDK optimizes user experience by auto-selecting the prime location for connectivity.
- Ideal for evaluation and development
- Up to 25 PCUs
- Unity and Unreal Engine Plugins
- Web and mobile SDKs
- Documentation, Samples and AI Bot
- No registration required
- 100 peak concurrent users included
- 0,29 EUR per additional peak concurrent user
- Traffic included
- Hosting included
- Unity and Unreal Engine Plugins
- Web and mobile SDKs
- Documentation, Samples and AI Bot
What is Peak Concurrent User (PCU) ?
Concurrent Users (CU) denotes the total number of users actively connected to the ODIN servers at any given moment, while Peak Concurrent Users (PCU) represents the zenith of this count over a monthly duration. With ODIN’s advanced dashboard, enterprises can monitor real-time user engagement and even set constraints on the peak value, ensuring consistent budget management and optimal server resource utilization.
Requiring a Custom Pricing Structure?
Should our standard pricing model not align with your enterprise’s unique requirements, we invite you to reach out. Our team is committed to crafting tailored proposals that seamlessly match your needs.
Download the ODIN server and gateway tools to seamlessly deploy ODIN servers at your preferred locations.
- Perfect for high-security sectors: military, healthcare
- Internet connection optional
- 12 months software updates included
- Windows, Linux, macOS support
- On-premise, AWS, Google Cloud, Azure, and more
- Horizontally scalable
- Community Support via Discord
- Documentation, Samples and AI Bot
- Ideal for custom deployments on your infrastructure
- Internet connection required
- Access to latest server downloads
- Windows, Linux, macOS support
- On-premise, AWS, Google Cloud, Azure, and more
- 1.000 concurrent users per instance (more on request)
- Horizontally scalable
- Community Support via Discord
- Documentation, Samples and AI Bot
Requiring a Custom Pricing Structure?
Should our standard pricing model not align with your enterprise’s unique requirements, we invite you to reach out. Our team is committed to crafting tailored proposals that seamlessly match your needs.
Premium Support Packages
Our Premium Support Packages are crafted to meet your specific needs, offering unparalleled, dedicated support to ensure a smooth and successful ODIN experience. Explore our options to align perfectly with your project support needs.
- 4 hours of dedicated One-on-One Support
- One-time payment
- 8 hours of dedicated One-on-One Support each month
- Dedicated Discord/Slack Channel
- Fast response within 24 hours
- Up to 40 hours of dedicated One-on-One Support each month
- Dedicated Discord/Slack Channel
- Fast response within 8 hours
- Hands-On Coding Assistance
- Custom feature implementation
What Others Say
ODIN was one of the best decisions we made regarding voice as it integrates with Unreal Engine’s built-in spatialization & occlusion systems, compared to competitors, this provided a much more immersive experience for our player-base. Their team was extremely helpful and quick in sorting out any issues we had during our setup process.
We have been the first to go live with ODIN onboard and what can we say? It was worth the risk, the ODIN integration was smooth and the ODIN dev team always supported us in the best manner. We are happy for the mutually beneficial and friend-like partnerships we share with 4Players.
ODIN is by far the best professional approach for a multiplayer cross platform voice chat system for your video game in-between Steam Decks, macOS and Windows.
I’ve been using ODIN since the beta release with a multiplayer VR game and it is working flawlessly! It’s very easy to set up and integrate into the project. You can do all sorts of cool things, it’s basically just an actor sound component that you can use and apply effects to like any other sound in Unreal. This is a 3rd-party service, it will work across your game servers in case you want that.
I have been working with ODIN both in Unreal and Unity for quite some time now and massively appreciate the easy integration and the flexibility it provides. I can adapt ODIN to any use case I can find, it’s massively scalable and it supports all the fancy features like 3D audio, audio occlusion and voice filters. From now on my first choice for any application that requires Voice Chat.
Experience Yourself
Download our samples and experience spatial 3D audio with friends, family and colleagues
Spatial Realism with ODIN
Experience the revolution in game communication with ODIN’s Voice Chat. Perfect for Unity, Unreal, and other 3D engines, our technology brings voice to life. Hear voices change with distance, direction, and in-game barriers, creating an unmatched realistic audio experience. Watch our video to see how ODIN transforms real-time voice chat, making it an integral part of your game’s world.