Use cases
We have compiled a couple of standard use-cases for ODIN and show you which benefits ODIN has to offer. So let’s get started:
Basic concepts
It’s important to understand the basic concept of ODIN:
graph BT
subgraph ODIN Server
OR2[Odin Room 1]
OR[Odin Room 2]
end
subgraph Mobile
ClientA[Client D] <--> OR
ClientB[Client C] <--> OR
end
subgraph Desktop
ClientB[Client C] <--> OR2
ClientC[Client B] <--> OR2
end
subgraph Consoles*
ClientD[Client A] <--> OR2
end
ODIN is a cloud-based client-server application that can easily be integrated into any game or application and even the web. All clients (i.e. users) connected to the same room can chat with all users in that room. Some users may not send any audio and just listening (i.e. spectator). Users also can connect to multiple rooms at the same time and it’s up to you to send audio to all rooms or just one of them.
Multiplayer games
With its 3D positional audio and deep engine integration ODIN is best suited for shooters and battle royal games. But also hide & seek games and many other multiplayer first person or third person games or even virtual reality and augmented reality video conferencing solutions.
Let’s consider a game with these features:
- Multiplayer shooter game
- Two gameplay modes: Team Based (like in CounterStrike or Rainbow Six Siege) and Deathmatch (last man standing)
- Private team chat: All users sharing a team can talk to other team members
- World chat. The voice and sound from players should also be “leaked” into the world.
- Lobby where players can hang around to find team members and to just chat
Now, we’ll have a look at the network topology of such a game. It might be a bit confusing first, but it’s basically very simple. We hope you read on if we say that you can implement these voice chat features production ready within one day - no registration required!
graph LR
subgraph ODIN Server
direction RL
OR3[Room Lobby]
OR[Room A_Team_A]
OR2[Room A_Team_B]
World[Room A_World]
World2[Room B_World]
end
subgraph Team A on GS A
PlayerB[Player B] <--> OR
PlayerB[Player B] <--> World
PlayerC[Player C] <--> OR
PlayerC[Player C] <--> World
end
subgraph Team B on GS A
PlayerE[Player D] <--> World
PlayerE[Player D] <--> OR2
PlayerF[Player E] <--> World
PlayerF[Player E] <--> OR2
end
subgraph Deathmatch on GS B
PlayerI[Player F] <--> World2
PlayerJ[Player G] <--> World2
end
subgraph Lobby
PlayerH[Player A] <--> OR3
end
subgraph Gameserver Fleet
GS[Gameserver A] --> PlayerB
GS[Gameserver A] --> PlayerC
GS[Gameserver A] --> PlayerE
GS[Gameserver A] --> PlayerF
GS2[Gameserver B] --> PlayerI
GS2[Gameserver B] --> PlayerJ
end
As you have learned in Basic Concepts all users connected to the same room will be able to talk to anyone else in that room. A room in ODIN is just a string. It can be anything and a room is created dynamically if the first player connects to that room and is deleted once the last user has left the room:
- You don’t need to do any book keeping
- Figure out a good algorithm to deterministically create a text string out of your game logic so that users that join the same room that should be able to chat together.
In this example, we use the string Lobby
for all players running the game but not playing yet. Of course, you could
have different rooms within the lobby like Welcome
and Finding_Players
.
Next, we have players B - E that are playing on Gameserver A in two different teams. For each team, a room has been
created which is a string concatenated from the Name of the Gameserver (could be an IP address and Port for example)
and the Team Name which is A_Team_A
and A_Team_B
. Just connect to that room like this and all team members will
be able to chat together.
OdinHandler.Instance.JoinRoom("A_Team_A");
The next thing we need to add is the world chat. Check out this image to understand what we mean with that:
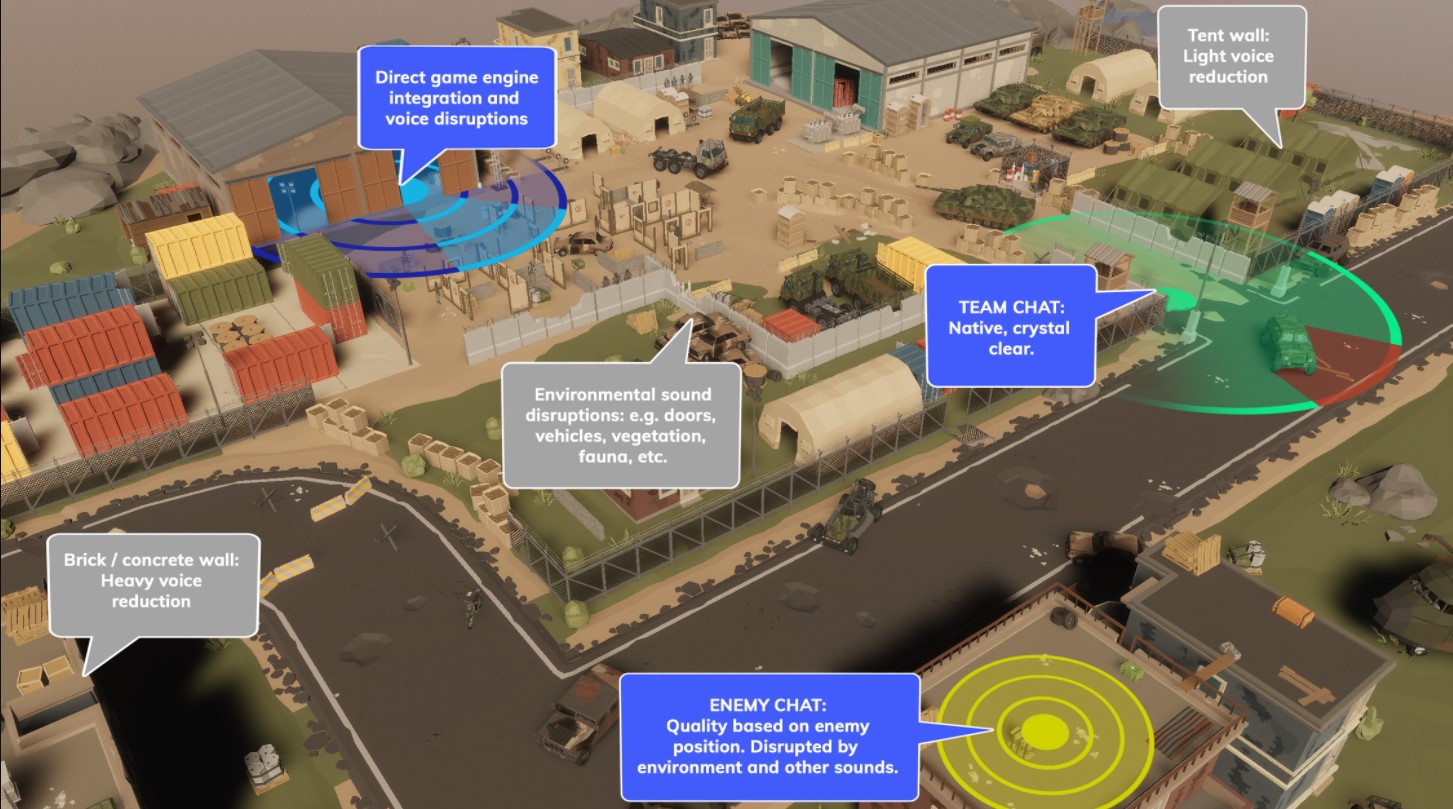
A game map showing ODIN integration
In real world, when people talk to other people via headphones, other people around can hear what that person is
saying. This is what we mean with “World chat”. You can just connect all players to another room called A_World
for the “world” they are in. And now, other players will be able to listen to what you are saying to your team
members. So, better don’t scream and make sure nobody is listening.
So, our code would look like this:
OdinHandler.Instance.JoinRoom("A_Team_A");
OdinHandler.Instance.JoinRoom("A_World");
And if someone changes the team, you’d use these two lines:
OdinHandler.Instance.LeaveRoom("A_Team_A");
OdinHandler.Instance.JoinRoom("A_Team_B");
The team based chat should not be 3D positional audio, because in real world the team members would have headphones
on while talking to their team members where volume is always the same. As we have a deep engine integration you can
just disabled 3D audio for these audio connections for example with one simple setting in Unity: spatialBlend
needs to be 0.0f
for these use cases and 1.0f
if you want to have 3D audio. That’s just
another one line of code to completely change the user experience!
Unity or Unreal do all the heavy lifting of 3D audio calculation for us. Game engines automatically do the 3D spatial audio effects for us. And we can use standard game engine audio features like mixers and DSP to create fancy audio effects like echo (in large rooms) or signal noise to make the players experience even more realistic.
Please note: ODIN can handle thousands of users within a single room and rooms can virtually scale indefinitely and adjust to the games load in real-time. Whatever you are planning. We got you covered. If you are interested in hosting ODIN servers yourself: That’s also possible.
Sounds interesting? Then get started for free and without registration:
MMOs
Massively multiplayer online games are very easy to implement with ODIN. You can have the same network topology as seen above for multiplayer games. As MMOs typically have huge worlds they often have some sort of segmentation.
We’d love to play a game like this:
- Space MMO
- Every solar system has its own gameserver (for segmentation) and players can jump from one solar system to another via worm wholes (i.e. does not take much time to transfer from one solar system to another)
- The clue is, that every player has a radio installed with 5 channels, and they can talk to all other players in that channel - independently of their position in the galaxy.
- Players can also call friends in a direct chat.
Let’s have a look at the network topology if this game (radio channels 3 - 5 omitted for clarity):
graph LR
subgraph ODIN Server
direction RL
C1[Room Radio_Channel_1]
C2[Room Radio_Channel_2]
DC[Room DirectChat_FG]
end
subgraph In Game
PlayerH[Player A] <--> C1
PlayerB[Player B] <--> C1
PlayerC[Player C] <--> C1
PlayerF[Player D] <--> C2
PlayerE[Player E] <--> C2
PlayerI[Player F] <--> DC
PlayerJ[Player G] <--> DC
end
subgraph Gameserver Fleet
GS[Solar System A] --> PlayerH
GS[Solar System A] --> PlayerB
GS[Solar System A] --> PlayerC
GS[Solar System A] --> PlayerF
GS2[Solar System B] --> PlayerE
GS2[Solar System B] --> PlayerI
GS2[Solar System B] --> PlayerJ
end
As you can see, ODIN is completely independent of your multiplayer topology. This allows you to quickly find the best approach of voice communication within your game without constantly refactoring your client server architecture. ODIN is a pure client side implementation and thus easy to implement!
In the diagram above we have this “game state”:
- Players A-D are in Solar System A, while players E-G are in Solar System B.
- Players D and E are talking in the same radio, although they are on different game servers and therefore solar systems.
- Players A-C talk in radio channel 1.
- Players F-G have a direct chat.
Direct Chat
For the direct chat feature we have created a simple room name with DirectChat_FG
, which is just a string
concatenated from the fixed keyword DirectChat_
and then the ID or name of Players A and Player B. Both players are
connected to that room like this (pseudo code):
class Player
{
private void GetPlayerID()
{
return netID;
}
public void CallPlayer(Player otherPlayer)
{
// Join direct chat room
string roomName = "DirectChat_" + this.GetPlayerID() + otherPlayer.GetPlayerID();
OdinHandler.Instance.JoinRoom(roomName);
// Signal the client of the other player to join the same room
otherPlayer.RoomJoinRequest(this, roomName);
}
public void RoomJoinRequest(Player otherPlayer, string roomName)
{
// Show "Ring Notification"
UI.ShowIncomingCallNotification(otherPlayer.name, roomName);
}
public void OnAcceptIncomingCall(string roomName)
{
// Signal the client of the other player to join the same room
OdinHandler.Instance.JoinRoom(roomName);
}
}
That’s it. It’s as easy as figuring out a good string, connecting to a room and then signaling the other client an incoming call. The other client is showing a UI with a button to accept the call. If accepted the other client joins the same room, and they can talk. Hanging up is just leaving the room!
Radio with different channels
This is easy to do. The Player has a UI where he can set the radio channel. For each radio channel we just create a
simple string like Radio_Channel_1
and Radio_Channel_2
. When a player changes the radio channel, we just need to
leave the current room and connect the other room:
class Radio
{
private string _currentRadio = null;
public void OnRadioChannelChanged(int channel)
{
if (_currentRadio != null) {
OdinHandler.Instance.LeaveRoom(_currentRadio);
}
_currentRadio = "Radio_Channel_" + channel.ToString();
OdinHandler.Instance.JoinRoom(_currentRadio);
}
}
Sounds interesting? Then get started for free and without registration:
Open World
Open world games are awesome, because they give gamers so much freedom, however, those are not so easy to implement. At least the voice part will be very easy to do.
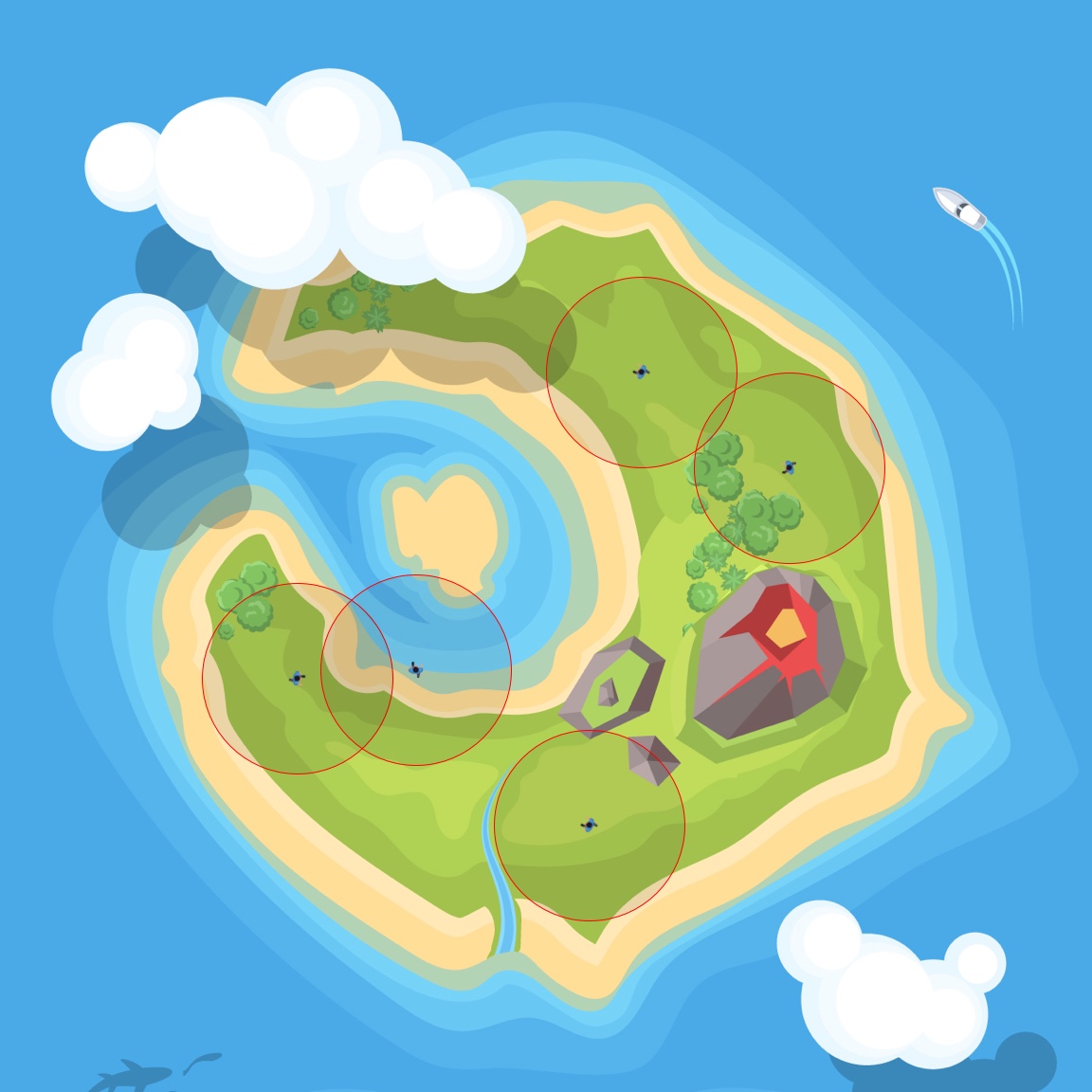
Open world with action circles
In this example, we have two players running around a huge map. ODIN can handle thousands of users in one room with ease. The deep engine integration of ODIN enables developers to leverage the skills they already have and don’t need to learn something new.
All you have to do is to “through” all players in the same room. ODIN servers know where the players are in 3D space and can route voice packets only to those players that are close enough. This way, CPU on client side is only spent on voice that happens nearby. Network bandwidth is also only used for the necessary audio packets with zero overhead.
Our deep engine integration does not require you to do anything? Just join a room on client side, handle a couple of callbacks and that’s all.
Volume will change with the distance between the players and with headphones or stereo speakers they’ll hear the direction where the other player is. It’s an immersive experience for your players!
Sounds interesting? Then get started for free and without registration:
VR and AR
VR games and applications need to be as immersive and realistic as possible to prevent users from getting motion sick and to feel strange when the headset is on. While this is of course true for graphics, it’s even more important for audio. Headset creators have spent a lot of efforts in creating good headphones and audio solutions for their devices, let’s make use of them!
Voice chat in VR (and to some extend in AR) is even more important than screen based gaming, as typing in VR is an awkward experience and voice is the only way to chat with other people efficiently. And it’s much more immersive, too.
Build virtual conferences, cinemas, multiplayer games and many more with ODIN and real-time 3D audio. In VR, performance is key, and ODIN with its deep engine integration is best suited for these kind of experiences.
Sounds interesting? Then get started for free and without registration:
Virtual Conferences / Metaverse
An important vision these days is the metaverse. The idea is to build virtual spaces that allow people to do everything in the virtual world that they can do in the real world. But without the constraints of the real world. Beaming yourself in seconds to every place you imagine: in our lifetime that will not going to happen, but in virtual world, that’s not an issue at all.
One key feature of the metaverse is audio chats. It’s key and core feature. And spatial 3D audio - i.e. the voice of someone standing nearby is loader than someone else a couple of feet away, and the voice is coming from the direction the person is related to the listener. These are the core features of ODIN and can be implemented in minutes.
The metaverse is the vision, virtual conferences for example is one very good example for that. Surfing web pages on virtual conferences is boring and the feeling of visiting other people and companies is not there. Virtual offices or conferences where people can run around having “virtual physical meetings” is one way to replicate a more physical presence. However, it must be natural. You should be able to chat with everyone you see, but you should also be able to have a discreet one-to-one chat with someone else. And without the hassle of managing contact lists, calling other users and all those complexities that come with standard voice chat tools.
In this example use-case we have built a virtual conference, featuring different rooms. Users are running around and can listen and talk to everyone they see. As it is in the real world.
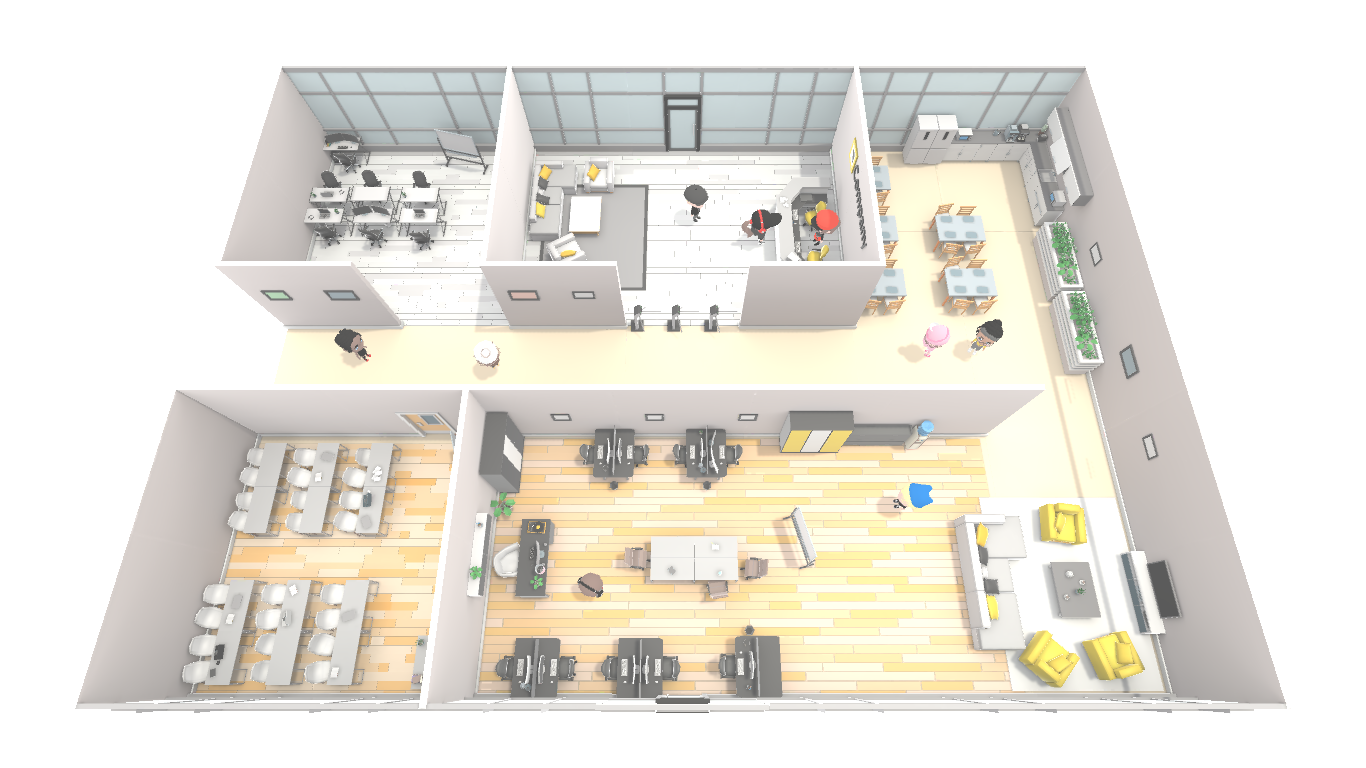
Virtual conference
We use ODINs spatial 3D audio. Every user joining the conference will join a global 3D ODIN room. This way, they can listen to the “noise” of other people talking and listening. Users can of course disable that room if they want to, however, the feeling of presence also includes some sort of noise and randomly hearing other people discussing and talking.
graph BT
subgraph ODIN Server
BR[Blue Room]
YR[Yellow Room]
FC[Floor Chat]
DC[Direct Chat A/B]
end
subgraph Members
ClientA[Member A] <--> DC
ClientB[Member B] <--> DC
ClientB[Member B] <--> FC
ClientD[Member D] <--> YR
ClientE[Member E] <--> YR
ClientF[Member F] <--> YR
ClientG[Member G] <--> FC
ClientI[Member I] <--> FC
ClientJ[Member J] <--> FC
ClientC[Member C] <--> BR
end
But, there are some benefits that don’t exist in the real world:
Silent direct chats. In noisy environments, it can be hard to have a direct chat. In our virtual world, two (or more) members standing on the floor can join a direct chat. They start talking in the public floor chat, then if they decide to have a private chat, one member invites the other member to join a private conversation, and if the other one agrees, they will join a private randomly created room. Users can still keep their connection to the floor chat (dampened with lower volume) so that they still hear what is going on outside and can react if someone reaches out to them, but they will be able to easily focus on the private conversation.
Next, we have virtual meeting rooms. These are spaces that have a separate ODIN room, that means, whenever you walk into that virtual space, the user will automatically join another ODIN room only available for those people within that virtual space.
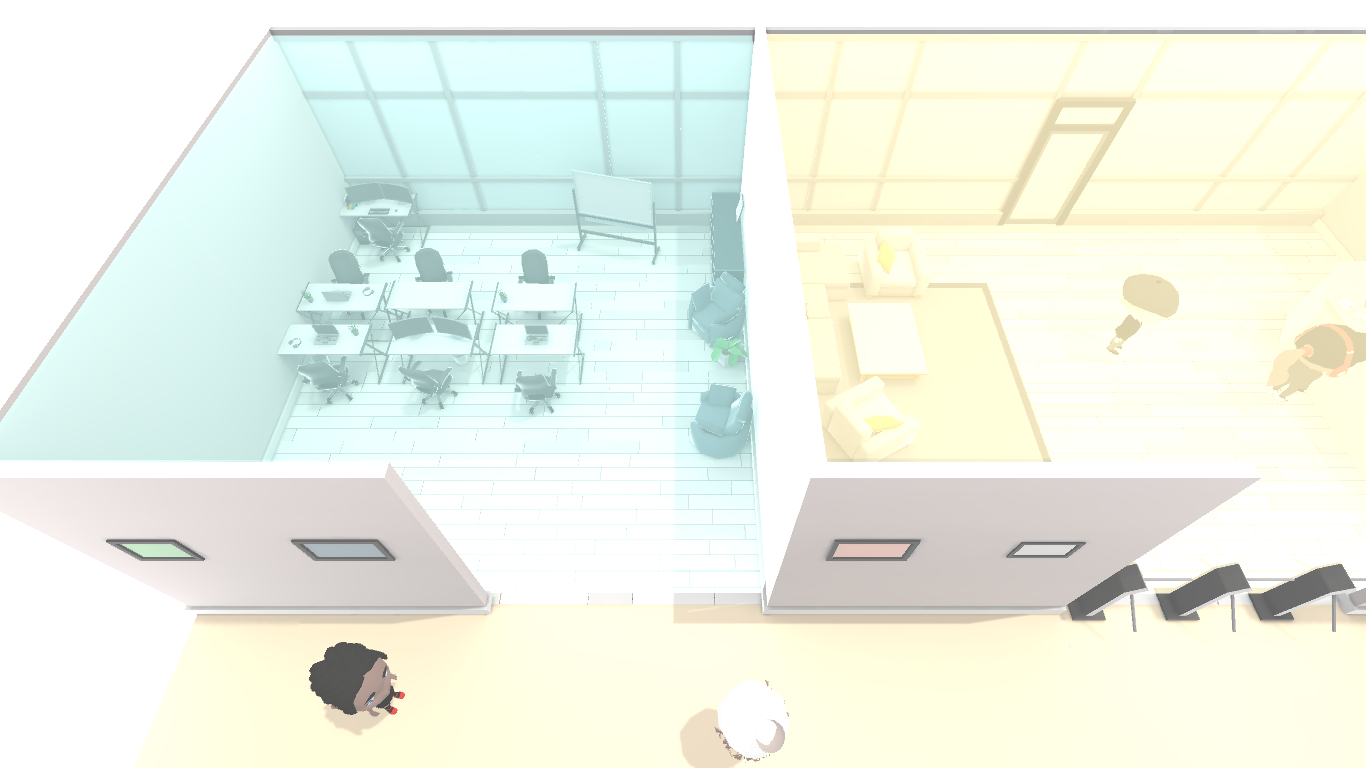
Virtual rooms with private audio sessions
In this example, we have that “blue” room and a “yellow” room. Every user walking into that space triggers a collider which then joins the room. Of course you can use ODINs access key feature to only allow specific users to join that room. It will be a secure connection and only those (unlike in the real world) that have the permissions will be able to listen to that chat.
Once users walk out of this space, they leave the ODIN room and will join the global 3D audio room again to participate in the public chat.
You can build these kind of applications with just a couple lines of code (pseudo code!):
class VirtualConferenceMember
{
public void Start()
{
// Join public floor chat
OdinHandler.Instance.JoinRoom("Floor_Chat");
}
public void OnTrigger(Collider collider)
{
if (collider.collision.direction == Collider.Direction.Entering) {
// Join private room and leave public "floor" chat
OdinHandler.Instance.JoinRoom(collider.name);
OdinHandler.Instance.LeaveRoom("Floor_Chat");
} else if (collider.collision.direction == Collider.Direction.Exiting) {
// Leave private room and join public floor chat
OdinHandler.Instance.LeaveRoom(collider.name);
OdinHandler.Instance.JoinRoom("Floor_Chat");
}
}
}
Sounds interesting? Then get started for free and without registration:
Singleplayer games
One use-case for Singleplayer games is “support”. Sometimes players get stuck at specific locations within the game. You can use our Web based framework to integrate ODIN into your web based community like your Forum. Within your game you could place a “Get help” button. If a player clicks on that button, the player will be connected to the same room as community members connected using the web app. The game can also leverage User Data to provide the exact level and location to the community, so they can quickly help! You can even create a simple bot, that listens on OnPeerJoined events and sends a push notification to all community members via web push notifications that are not currently joined to the ODIN room.
graph BT
subgraph ODIN Server
OR[Odin Room]
end
subgraph Game
ClientA[Player A] <--> OR
end
subgraph Web App
ClientB[Community A] <--> OR
ClientC[Community B] <--> OR
ClientD[Community C] <--> OR
end
subgraph Notification
Script[Bot] <--> OR
end
Sounds interesting? Then get started for free and without registration: