Input Device Selection
Unreal does not provide an easy method to switch input devices (like a microphone) when using the default Audio Capture, even though most players would expect this feature in a game with Voice Chat. Let’s take a look at how we handle this in the ODIN plugin.
By David Liebemann at SciCode Studio.
Creating an Odin Audio Capture
To change input devices, instead of using the default Create Audio Capture
method, we’ll use the Create Odin Audio Capture
function call. This will create an extended Audio Capture object, that allows us to change the audio capture device using either Blueprints or C++.
Your logic for joining an Odin room should now look something like this:

Blueprint for joining the Odin room
Please note, that we store a reference to the Odin Audio Capture
object as a variable - we’re going to use it in the next sections to display the available devices in the UI.
UI Creation
You’ll probably have to adjust the next steps to fit your UI implementation, but in general it’s easy to adapt the necessary UI elements.
We’ll create a new Blueprint Widget that gets displayed when the player presses `tab. This Widget should show the available capture devices in a drop-down box and change the active device on selection.
- Create a new Widget Blueprint in your project - we’ll call it
BPW_SelectInputDevice
. - In the widget’s event graph, create a new Custom Event called
UpdateAudioCapture
with an input parameter of typeOdin Audio Capture
. We’ll use this event in the next section, so simply leave it unconnected for now.
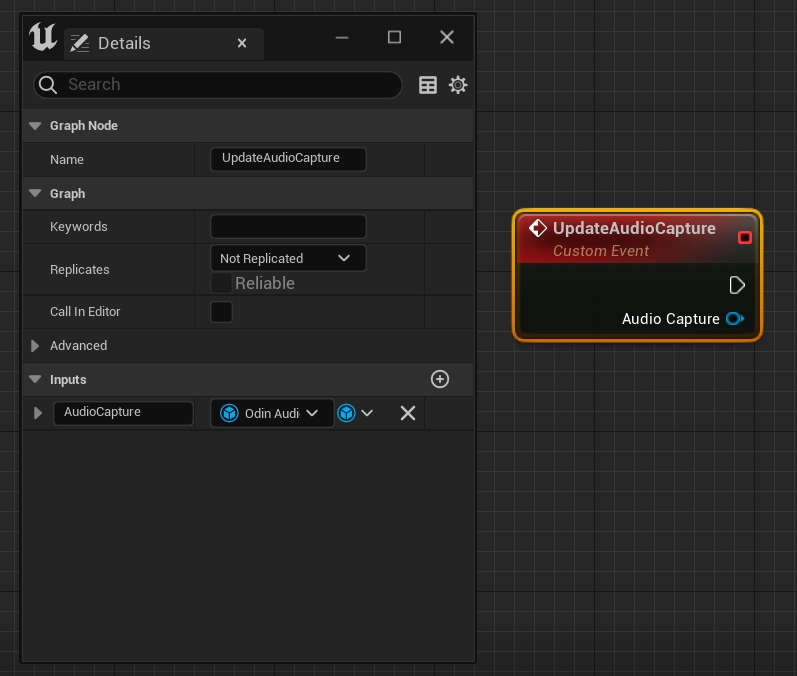
The Custom Event UpdateAudioCapture
in the Widget Blueprint
In your player controller’s
Begin Play
, add aCreate Widget
call, select the classBP_SelectInputDevice
, connect theSelf
property to theOwning Player
input and save the resulting widget object in a variable.Initializing the device selection widget in the player controller.
The final step in the player controller is to add logic for toggling the “Select Input Device” UI, as shown below. Please note the call to
Update Audio Capture
when making the UI visible. This will supply theAudio Capture
object to the widget blueprint, which we’ll use to request the available capture devices.Toggle the “Select Input Device” UI based on Input mapping.
If you can’t create the InputAction ToggleDeviceSelection
event, make sure to add an Action in Project Settings > Input > Action Mappings
and bind it to a keyboard input - we’re using Tab for testing.
Widget implementation
Currently the widget does not contain any visible UI elements, so let’s change this. We’ll add a simple see-through background and a dropdown box, in which we’ll list all available capture devices. Changing the selection in the dropdown box will change the capture device used for Odin. Additionally we’ll preselect the currently active capture device in the dropdown box.
In the widget’s
Designer
mode, add aCanvas Panel
as the root element and aBorder
andComboBox (String)
as direct children. Set theBorder
element’sBrush Color
to(0,0,0,0.5)
to make it darken the gameplay, but still keep it see-through. Additionally, let’s give theComboBox
a meaningful name - e.g. “SelectionDropBox”.The widget’s hierarchy and display after adding all elements.
Let’s now switch to the
Graph
mode and start extending our Custom EventUpdateAudioCapture
. We’ll store theOdin Audio Capture
object as a variable and callAsync Get Capture Devices Available
. This will return the available capture devices and the current device as outputs in the connected event.Request the available capture devices.
The Odin Audio Capture
object also contains synchronous function calls to access and change capture device data. During testing we’ve noticed that these synchronous calls can take quite a while to run: more than 100ms on average, in some cases more than a second. This lag is due to Unreal’s internal implementation for changing capture devices. We therefore strongly recommend using the async function calls.
Let’s name the connected event
OnReceivedDevices
. We’ll store a reference to the available devices, as well as retrieve and store the current device name from theCurrent Device
Info structures.Because
OnReceivedDevices
will be called each time we open up the UI, we’ll need to reset the current options by callingClear Options
on theSelection Drop Box
.Now we need to add the available capture device names as options in the dropdown box. We iterate over our
Available Devices
array, retrieve theDevice Name
from the device info structures and add that as an option to theSelection Drop Box
.Afterwards we’ll preselect the currently active device by calling
Set Selected Option
with ourCurrent Option
. Your blueprint logic should look something like this:Filling the dropdown box with available capture device options.
Finally we’ll change the capture device, when the user selects an item from the dropdown box. Add the event
On Selection Changed (SelectionDropBox)
to your Event Graph. TheSelected Item
will contain the name of the input device that should become the active capture device. Let’s do a quick check for whether theSelected Item
is empty or equal to the currently selected option. If the item passes the checks, we callAsync Change Capture Device by Name
with theSelected Item
as input. TheOn Change Completed
callback will let us know, whether the given device was activated successfully. Your blueprint should look something like this:FChange the capture device on selection.
Now anytime a user chooses a new option, the Odin Audio Capture
object will change the capture device and restart the input stream automatically.
What’s next?
This is a very simple implementation with minimal UI, which you’ll be able to easily adapt to your project’s needs. For more information on Unreal with Odin Voice Chat, check out our Discord and take a look at the following guides we’ve prepared for you: